Generate label footprints for Satellite Imagery: the Pythonic Way [Part 3]
Published:
Now that we have got the labels, we can want to see whether they are correct or not. You can easily do so by visulizing the labels on Google Maps! We will use a Python visualization library called Bokeh for this. This is a pretty easy thing to do!
Things to know or to brush up
- Bokeh. Get to know about this Python visualization library here.
Before we start, go ahead and get you Google Developer Key. This is required for this part of the tutorial series. The code can be found in this notebook.
import fiona import numpy as np from bokeh.models import ColumnDataSource, HoverTool, LogColorMapper from bokeh.palettes import RdYlBu11 as palette from bokeh.plotting import figure, show, save from bokeh.io import output_file, show from bokeh.models import GMapPlot, GMapOptions, ColumnDataSource, Circle, Patches, MultiLine, Oval, DataRange1d, PanTool, WheelZoomTool, BoxSelectTool
dataframe['x'] = None dataframe['y'] = None for index, row in dataframe.iterrows(): x = [] y = [] for pt in list(row['geometry'].exterior.coords): x.append(pt[1]) # lat y.append(pt[0]) # lon dataframe.set_value(index, 'x', x) dataframe.set_value(index, 'y', y) # Drop the 'geometry' column and create # ColumnDataSource for Bokeh g_df = dataframe.drop('geometry', axis=1).copy() gsource = ColumnDataSource(g_df) map_options = GMapOptions(lat=45.5, lng=-73.5, zoom=11, map_type=map_type) plot = GMapPlot( x_range=DataRange1d(), y_range=DataRange1d(), map_options=map_options) plot.title.text = "AOI" plot.api_key = key if shapely_type == 'Polygon': shape = Patches(xs='y', ys='x', fill_color="blue", fill_alpha=0.9, line_color=None) if shapely_type == 'Point': shape = Circle(x='x', y='y') if shapely_type == 'LineString': shape = MultiLine(xs="x", ys="y", line_color="#8073ac", line_width=2) plot.add_glyph(gsource, shape) plot.add_tools(PanTool(), WheelZoomTool(), BoxSelectTool()) show(plot) output_file("show_on_map.html")
- There are a bunch of import statements.
- Loop through the dataframe obtained from the previous tutorial. We have already saved that! Extract the (longitude, latitude) pair of each Polygon and that in a new dataframe columns. Here x and y p
- We remove the geometry column as it is no longer required and add in the newly created x and y columns into the dataframe. Create ColumnDataSource which is a format used by Bokeh to store the dataframe.
- GMapOptions(lat=45.5, lng=-73.5, zoom=11, map_type=map_type) helps locate the centre of the map that we are interested in. Here, these are the coordiantes of Montréal. The data will be overlayed in its vicinity.
- Next, we create an empty map object and provide our Google API key (which is a string).
- Specify the type of object. For buildings as we already know it is Polygon. Prettify the map with some tools to scroll and zoom in. And display the overlayed labels on top of the maps. Save it as a html file for future!

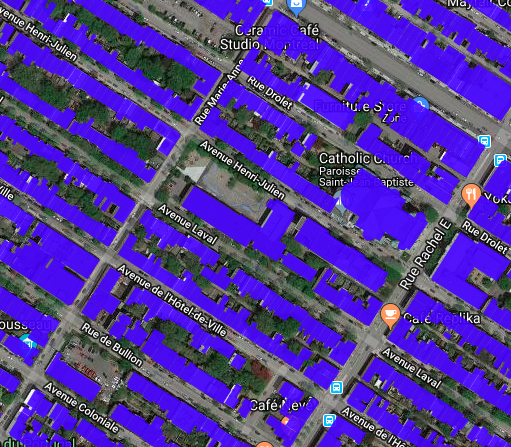
That's a wrap to our three part tutorial series. We learnt a lot and I am sure that you enjoyed the journey as much as I did both while working on this project and writing these tutorial blog posts. We learnt to query the OSM web API, use a python wrapper to do it and visulize the labels as well. We got a really nice hands on experience of making our own easy to use API. All the code, jupyter notebook, images, plots is located in this git repository. See you next time!
Credits and References
- The code was written during my internship. I should give credits to Planet and all the other cool open source projects and libraries/APIs that were used to make this possible. If you feel that I have missed some credits, feel free to email me and I will make sure they appear here.
- http://schiff.co.nz/small-towns/